Requirement
We’re building an application and we want a reasonably robust, quick-to-configure authentication service. We’ve started testing the water with other Azure services and we thought we’d evaluate Active Directory B2C (Azure AD B2C).
Context
We have a PAYG Azure subscription.
Tenants
From https://docs.microsoft.com/en-us/azure/active-directory/develop/active-directory-howto-tenant#use-an-organizational-azure-subscription
An AD tenant is… representative of an organisation. So it stands for a company. A tenant is a dedicated instance of the Active Directory service. A tenant houses user information about an organisation, including permissions etc.
To allow Azure AD users to sign in to your application, you must register your application in a tenant of your own. Publishing an application in an Azure AD tenant is absolutely free. In fact, most developers create several tenants and applications for experimentation, development, staging, and testing purposes. Organizations that sign up for and consume your application can optionally choose to purchase licenses if they wish to take advantage of advanced directory features.
Um… “you must register your application in a tenant of your own”? I’ll have to get back to you about what that means.
At the moment I’m confused about whether I can use my existing AD tenant – passively created when I signed up for Azure, and which I later linked to our company’s domain, almostalldigital.co.uk … simply by adding AD B2C to it; or whether I need to create a new tenant for that. In which case, can I use the same domain for the new tenant?
In the comments BTL in that docs page:
> In fact, most devs create several tenants and applications for experimentation
Really? is it that easy to create a tenant from scratch? I can have multiple tenants?
MS account replies:
yes and yes, but this assumes you have an Azure subscription already, and a user account that has been given admin access to that subscription (see https://docs.microsoft.com/azure/billing/billing-add-change-azure-subscription-administrator).
Once you have admin access:
– log in to the Azure portal (https://portal.azure.com) using your admin account
– select the “Create a resource” plus/+ option at the top of the left-hand pane
– select the “Security + Identity” option
– select “Azure Active Directory”
– specify an Organizational Name and unique Initial Domain Name
– select “Create”
The new basic tenant will be created with your Azure sign-in account as the sole member of the “Global Administrator” directory role, allowing you to administer it.
Hmm, OK. So that gives me a tiered exploratory approach, in descending order of optimism/ambition:
- Add AD B2C to my existing tenant, so I can register an application on almostalldigital.co.uk domain. I’ll try it but I’m worried that, having set up Active Directory for my @almostalldigital.co.uk users, it won’t let me set up AD B2C.
- Create another tenant, also for almostalldigital.co.uk, that’s separate, and configure AD B2C on that tenant.
- Use some other domain I own, create a tenant for that domain, and register an application that lives in that domain.
NB In reality what I achieved was sort-of like (2), but not really: I couldn’t use the almostalldigital.co.uk domain in the configuration of a new tenant… I ended up with a domain like aadtesttenant1.onmicrosoft.com, as you’ll see… below.
The experience
One of stumbling, eventually (via trying to configure AD B2C in the Azure portal) onto this:
https://azure.microsoft.com/en-gb/trial/get-started-active-directory-b2c/
1 Creating an AD B2C Directory
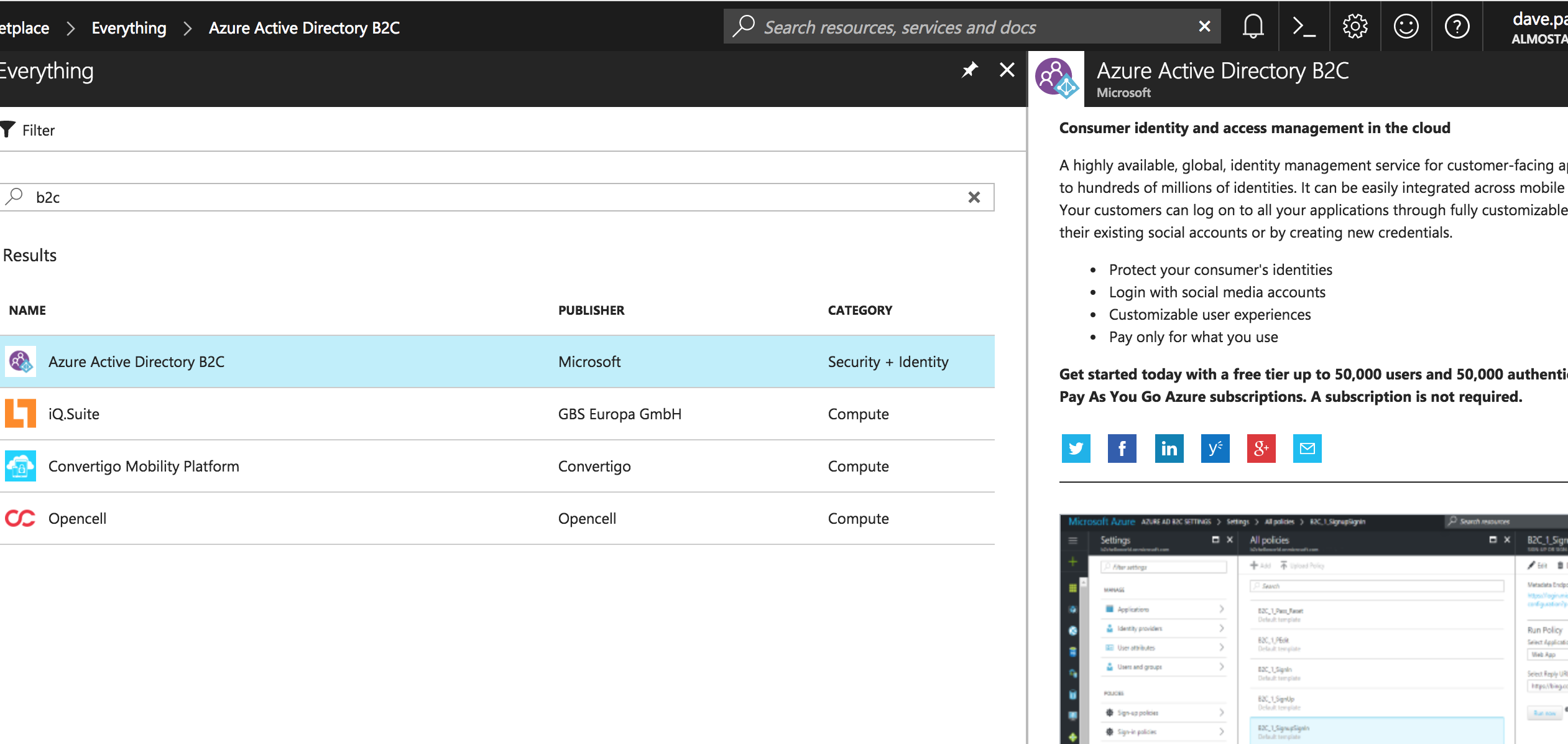
I click the New button at top left of the Azure portal and, in the huge list of things I could create a new one of, search for b2c.
I click on the option to create a new Azure Active Directory B2C.
2 Creating a new tenant
At the bottom of the right-hand panel is a blue button that says Create.
I click it.
Now I see a screen with options to create a new Azure Active Directory B2C tenant, or link an existing tenant to my subscription.
I haven’t ever set up a tenant before, so I decide to try creating a new one. I click that option and on the right-hand side I see a configuration panel:
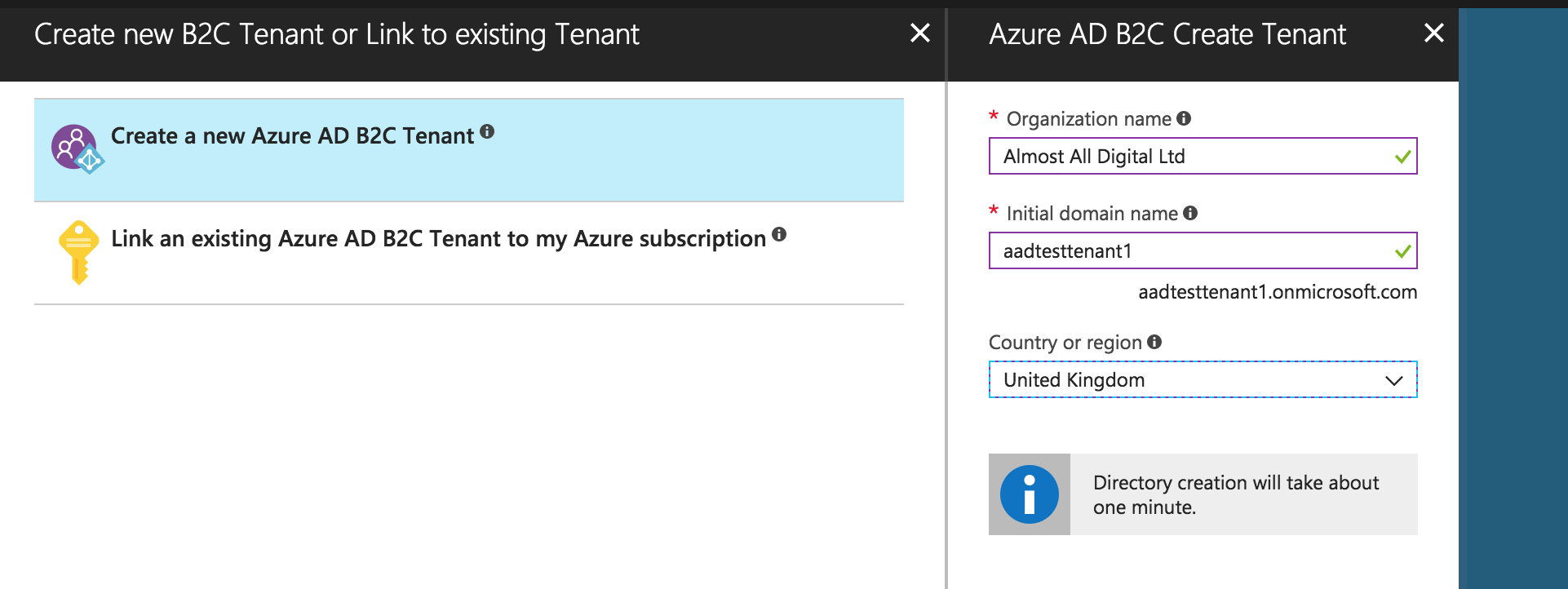
I stumble briefly because I try to set the Initial domain name to almostalldigital.co.uk. The error message says “invalid characters”… it wants a prefix to a *.onmicrosoft.com domain.
I’m slightly surprised for a moment that aadtest and aadtesttenant have both already been used… but presumably there are developers and IT staff all over the world doing the same thing, so I go with aadtesttenant1.
Somewhere, a handful of threads on a containerised server do some work for about a minute:
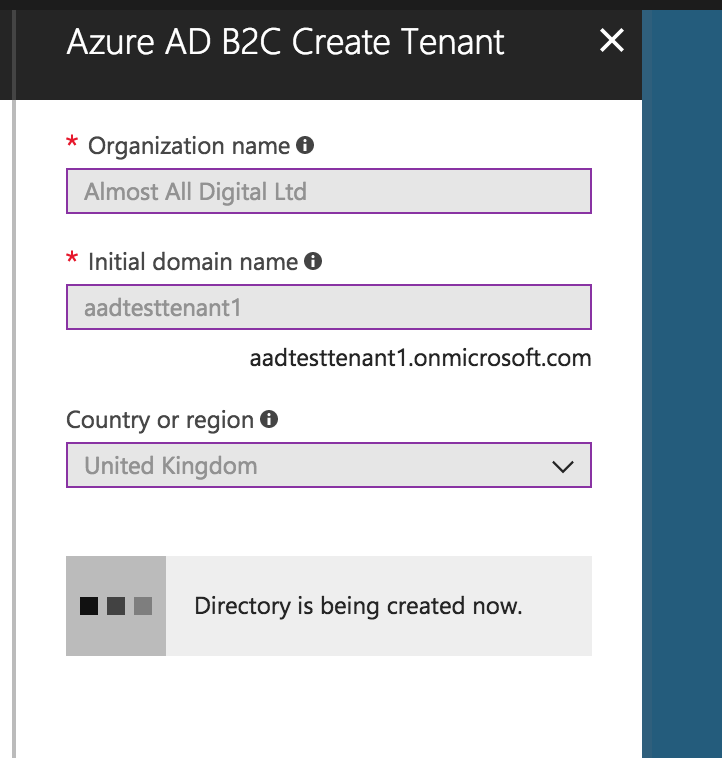
3 A moment of optimism
Then I see:
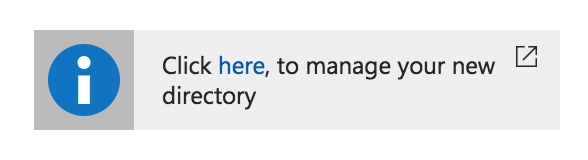
I allow myself to be optimistic. Only for a moment: the puff of dopamine barely registers as a warming in the flavour of my consciousness. But somehow, the Internet picks up on it, perhaps through unpublicised monitoring of the capacitance of my fingertips through my laptop’s replacement keyboard, because next I see:
4 This rubbish
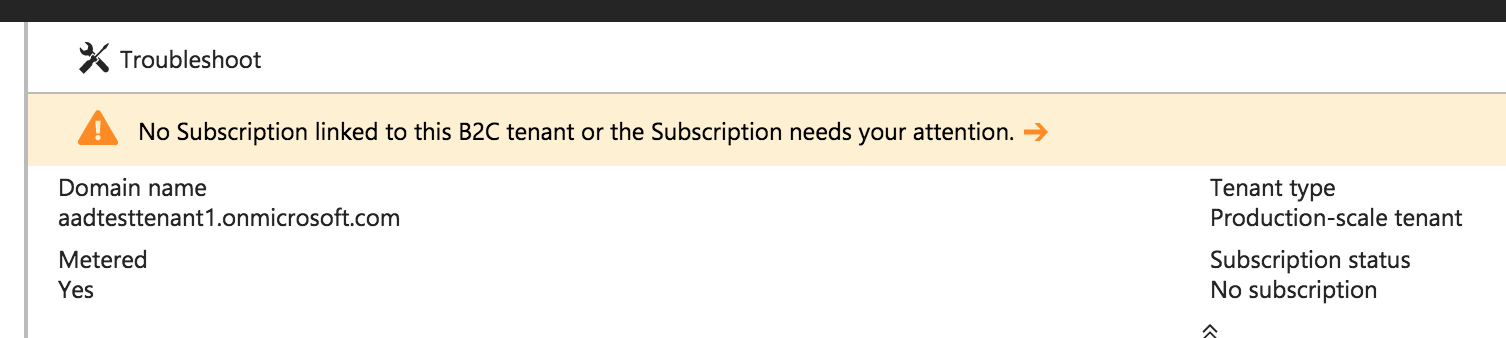
I am disappointed, because I began this process by logging in as an administrator user of an Azure subscription, and worked through the preceding steps.
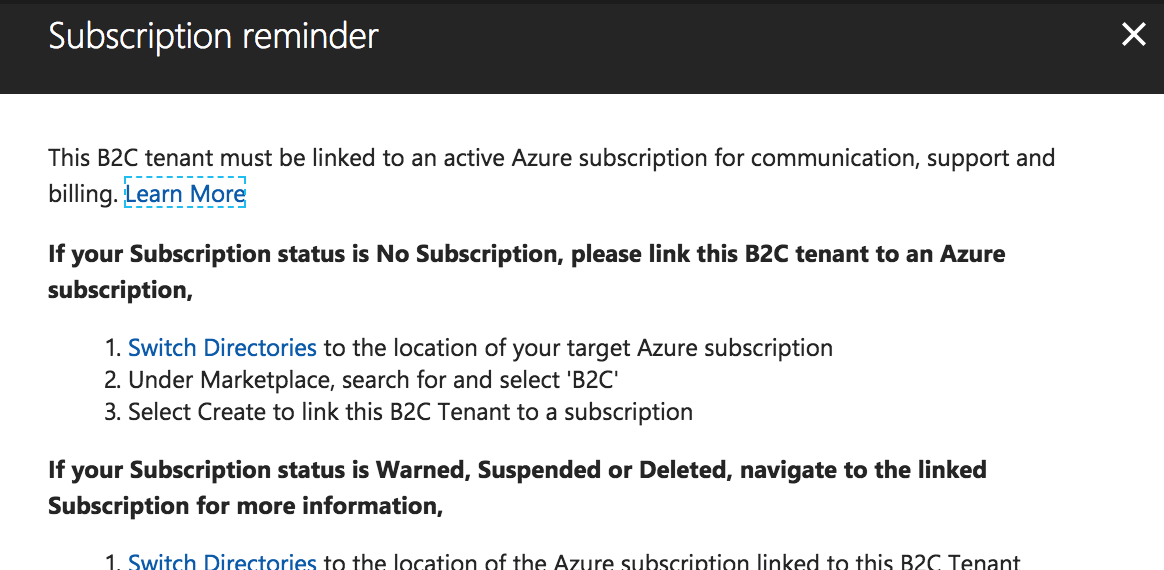
Oh: in step 3, when I clicked here, the link opened a new browser window, and I tutted because that meant more browser windows, and I closed my existing browser window. I should have rejoiced instead, because the new browser window probably meant that the information I was to view related to a new, different directory. Directory as in, instance of Active Directory as a service.
God, why is configuring a pay as you go federated login and permissions system for a web application running on a completely different hosting platform, and that will scale effortlessly to 100s of 1000000s of users worldwide so DIFFICULT?
5 Microsoft’s new Switching Directories experience
I’m going to click on the Switch Directories link from section 4, and see what happens…
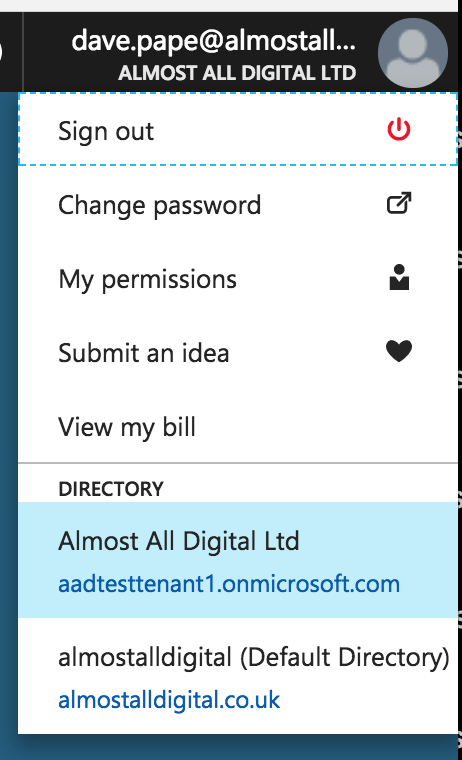
Ah – it triggers the same outcome as clicking on my username in the top right-hand corner of the screen: lots of options to do with my account… and now a list of Directories I could choose between.
They’re confusing to read, because I named my new Directory “Almost All Digital Ltd” which seems more official than the default Directory I set up by initially creating my Azure account. Perhaps I’ll rename it later.
I click on the default Directory… and start again, effectively, from step 1.
But when I see
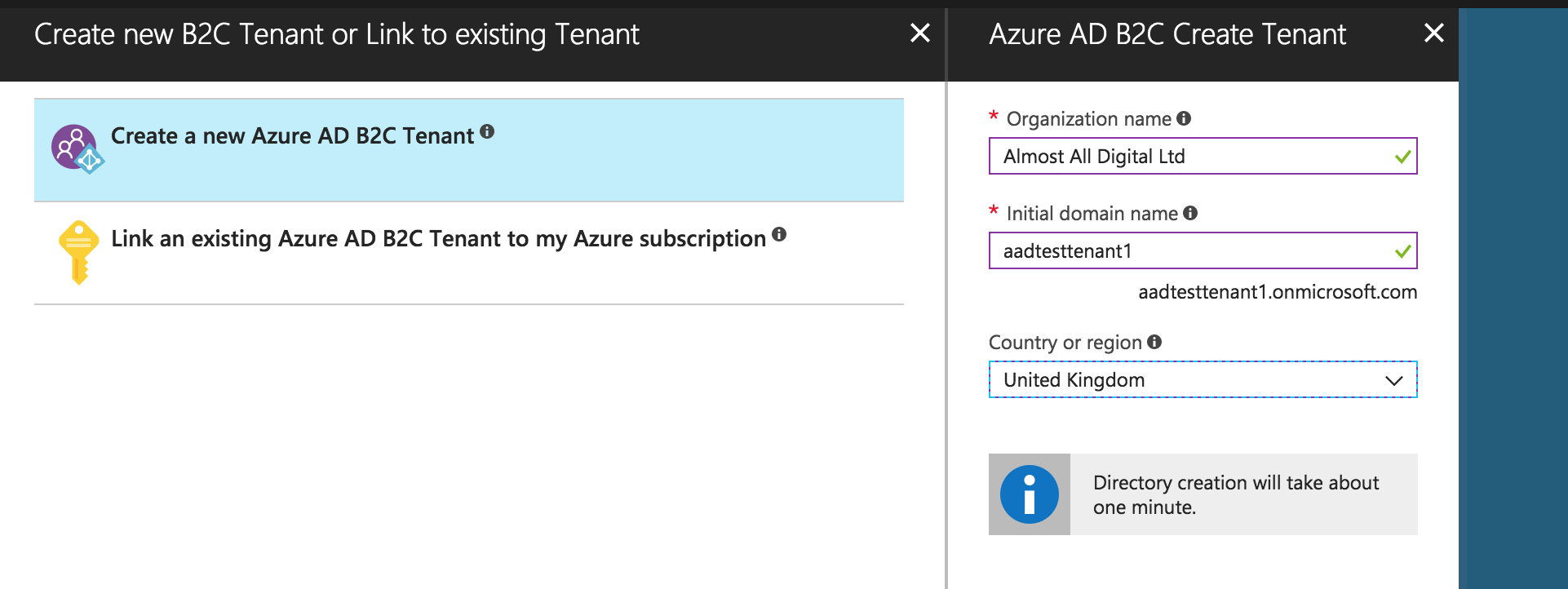
At this point… it’s subtle, and maybe points to a hint of over-literal thinking in the way my mind works, but I feel a slight wrongness, because I think of this screen as “the screen you use to create a new AD B2C Tenant”. But no, look, it’s perfectly clear – it’s labelled “Create new B2C Tenant or Link to existing Tenant“. What part of or Link to existing Tenant did I not understand?
I click Link an existing Azure AD B2C Tenant to my Azure subscription.
6 Configuring the link between my Azure subscription and the new Azure AD B2C Tenant I just created
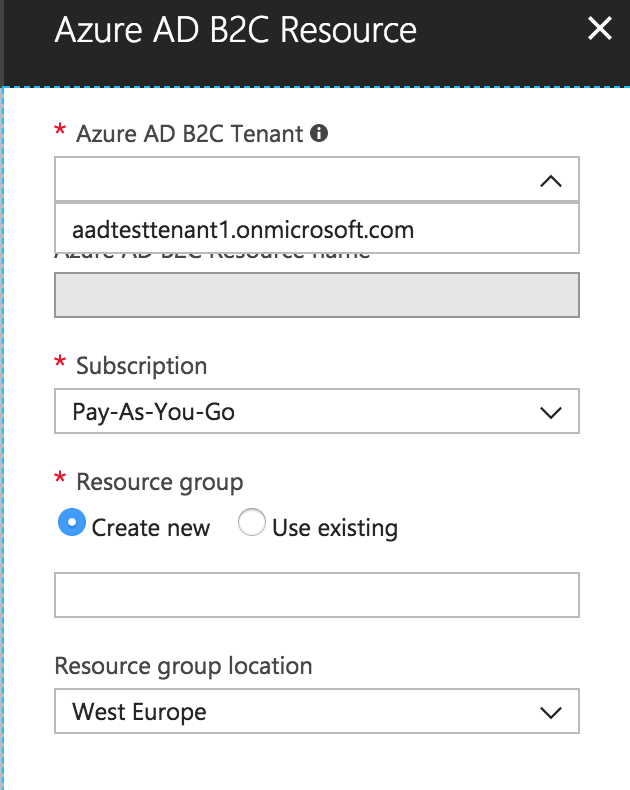
Another puff of dopamine, albeit less purely warm than the last: a panel where it seems I can configure the link between my Azure subscription and the new Azure AD B2C Tenant I just created. And the tenant appears in the dropdown menu of available tenants.
I try the following:
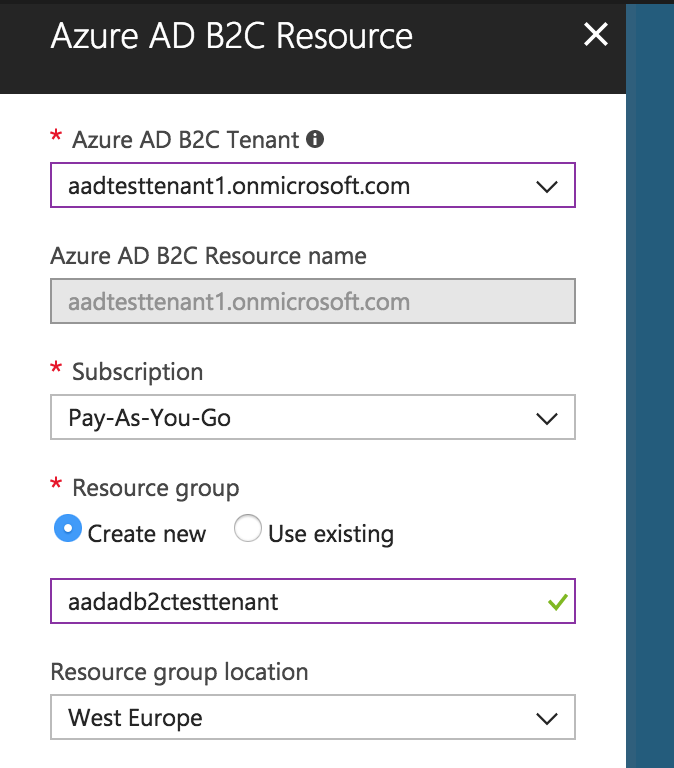
7 Success === anticlimax
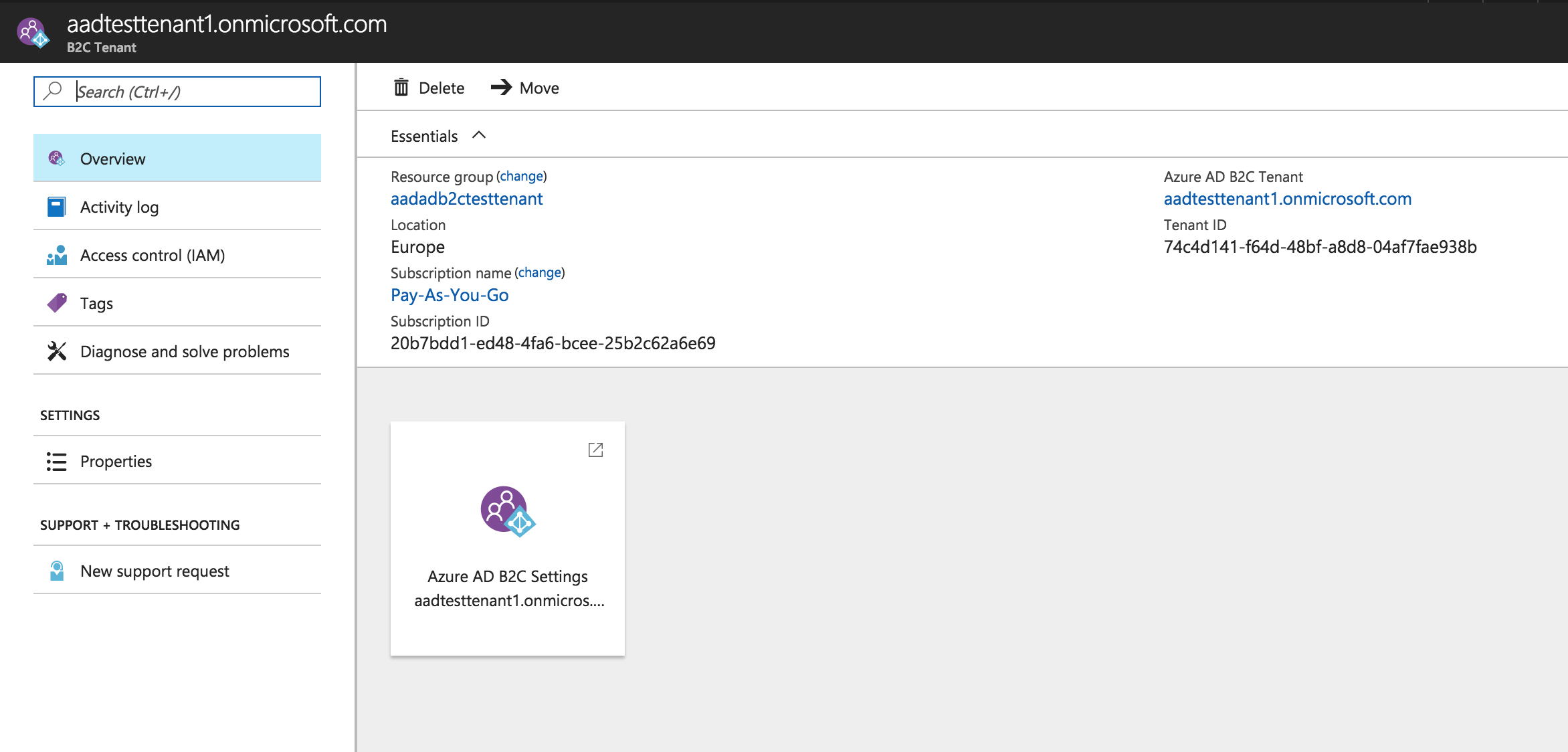
I think everything has worked, as far as it goes; but now, I can’t see how to do the following:
- Integrate the authentication service with an application on almostalldigital.co.uk
- Add custom user roles (I feel like, in the fullness of time, I’ll need roles like “Customer”, “Moderator”, “Support” etc)
Maybe that’s OK: maybe stepping through that 7-step process 1.857143 times is the first leg of my journey. I watched a video earlier today where a Microsoft product manager repeatedly referred to logging in as a user “journey”, and signing up as a different “journey”. So, perhaps there’s a three-masted ship anchored in the bay, silhouetted against the light of dawn, and I’ve just pushed a rowing boat off the sand into the shallows. Maybe now I can row to the ship; maybe then I can climb a rope ladder up to the deck; maybe then I can plot a course; maybe then I can set off on a year long journey South, around the Cape, passing the Gulf and eventually landing in a metaphorical Asia, where lies the answer to the challenge of adding a login screen to some fucking web page I wrote.
Coming up: Part 2